Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 에러
- MuchineRunning
- 면접
- 메타마스크
- 반도체 엔지니어
- 이더리움
- 반도체
- memory
- 던전앤파이터
- 던파
- 머신러닝
- 컴퓨터구조
- 네오플
- 유니티
- 게임기획
- Unity
- 아두이노함수
- 메모리
- 네트워크
- 인터럽트
- neople
- 아두이노
- 반도체 취업
- 유니티에러
- 네트워크보안
- MLAgent
- 보안
- 암호화
- 레지스터
- 아두이노우노
Archives
- Today
- Total
Dreaming Deve1oper
[Unity 3D] 오브젝트 중력작용 본문
이전 포스팅에서 방향키를 통해 오브젝트를 제어하도록 하였다.
이번 포스팅에선 오브젝트에 중력을 적용시켜보도록 하겠다.
https://juhuyunjjung.tistory.com/84?category=1029878
[Unity 3D] 방향키로 오브젝트 제어하기
C# Script를 통해 Object의 움직임을 제어하는 방법을 포스팅해보도록 하겠다. 우선, Character Controller Component에 대해 먼저 알아보도록하자. Character Controller는 유니티에서 캐릭터의 제어를 위해 제공..
juhuyunjjung.tistory.com
영상을 확인해보면, 오브젝트가 오르막길을 올라간 후 떨어지려고 하지만 그대로 공중에 떠 있는 것을 볼 수 있다.
그 이유는 다음과 같다.
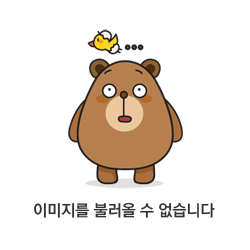
Character Controller Component는 충돌 처리만 가능할뿐 중력 처리는 불가능하기 때문이다.
이전 포스팅을 확인할 필요 없이 그냥 첨부된 코드를 복붙해도 무관하다.
작성한 스크립트는 오브젝트에 Add Component에 drag & drop 해준다.
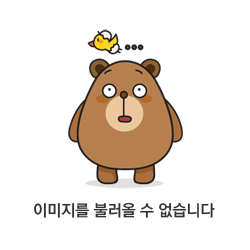
중력 작용 위한 스크립트를 작성해보도록 하겠다.
이전 포스팅에서 진행한 코드에 살을 붙여 진행하도록 하겠다.
Movement3D 스크립트
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Movement3D : MonoBehaviour
{
[SerializeField]
private float moveSpeed = 5.0f;
private Vector3 moveDirection;
private float gravity = -9.81f;
private CharacterController characterController;
private void Awake()
{
characterController = GetComponent<CharacterController>();
}
private void Update()
{
if (characterController.isGrounded == false)
{
moveDirection.y += gravity * Time.deltaTime;
}
characterController.Move(moveDirection * moveSpeed * Time.deltaTime);
}
public void MoveTo(Vector3 direction)
{
moveDirection = new Vector3(direction.x, moveDirection.y, direction.z);
}
}

PlayerController 스크립트
코드는 역시 아래를 참고하면 된다.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerController : MonoBehaviour
{
private Movement3D movement3D;
private void Awake()
{
movement3D = GetComponent<Movement3D>();
}
private void Update()
{
float x = Input.GetAxisRaw("Horizontal");
float z = Input.GetAxisRaw("Vertical");
movement3D.MoveTo(new Vector3(x, 0, z));
}
}
코드를 적용시키면 다음과 같이 중력 작용을 받아 오브젝트가 추락하는 것을 확인할 수 있다.
다음 포스팅에선 점프 기능을 구현해보도록 하겠다.
출처: https://www.youtube.com/watch?v=NQg1_NVi-6o
'유니티' 카테고리의 다른 글
배경음악/BGM/노래 첨부하기 (0) | 2022.02.03 |
---|---|
[Unity 3D] 점프 기능 추가하기 (0) | 2022.02.03 |
[Unity 3D] 방향키로 오브젝트 제어하기 (0) | 2022.01.25 |
Meterial을 통해 오브젝트 색깔 바꾸기 (0) | 2022.01.24 |
설치된 Visual Studio에 Unity 개발 환경 추가하기 (0) | 2022.01.23 |
Comments